Hello, if you have any need, please feel free to consult us, this is my wechat: wx91due
COSI 10a (Fall, 2024)
Introduction to Problem Solving in Python Programming Assignment #2
Program Description
For this assignment you will write a program for each of the following 3 problems and name each script Problem1.py, Problem2.py, etc.
You may talk to other students about the assignment and consult the slides and online resources for syntax, but all code you submit must be your own. Do not copy code directly from other students or from any online resources. The best way to not get caught plagiarizing is to not do it in the first place. The graders will be receiving a solution generated by ChatGPT to see how it will utilize style – so we highly recommend not getting your answer from ChatGPT. These assignments are training your ability to deconstruct problems and process solutions in code, not to see how quickly you can solve it!
Requirements
- All three programs must run without error and give the correct output.
- Your code must be commented and have docstrings, as well as descriptive function and variable names.
- There should be no code outside of a function except for a call to main().
- Your code should be well structured and split into appropriate functions.
- Include a comment at the top of each submitted file with your name, the assignment number, the program number, and a brief description of what the program does. For example:
#Full Name#COSI 10a Fall 2024#Programming Assignment 2#Problem 1#Description: …
You should limit your code to use only what we have learned through lecture 9, as well as topics covered in lectures 12 and 13. You should not use anything from lectures 10 and 11 (if statements, while loops).
Submission and Grading
There are 3 possible grades you may receive for this assignment: fail, pass, and pass+. Your program will be graded pass/fail on each of the requirements listed above. If your code does not meet requirement 1 (correctly named, produces the desired output without error) you will not receive an overall passing grade. Of the remaining requirements, you may fail no more than 1 to receive a passing grade. Submissions that meet all of the requirements will receive a grade of pass+.
There are 3 total problems in this assignment, please see them below.
Problems
Problem 1
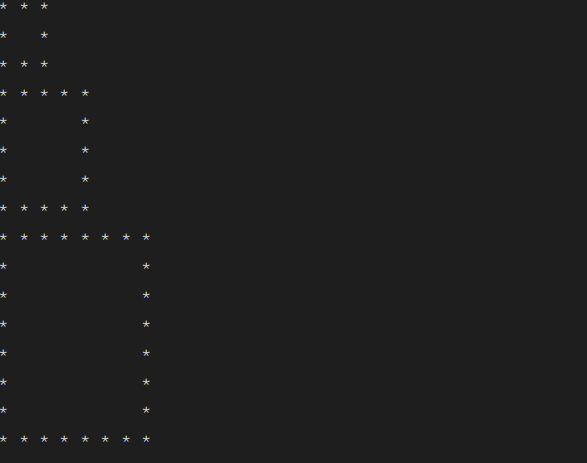
Problem 2
Problem 3
The Bank of Brandeis, NA offers 0.65% interest on savings accounts (yes, a very generous bank!), compounded annually. Create a program that allows a user to enter the number of years they want to invest with a specific annual deposit. Your output should look exactly like the following:
- The input for both should work for any number above 1. We will be testing this logic on many different use-cases.
- Exact spacing is negligible, but it should look very similar to the above output with exact logic.
- To achieve the rounded numbers you can use the Python round function. Say we wanted to round 105.3434 to 2 decimal places, we would do: round(105.3434, 2), where the latter number specifies significant figures.